A new/old look, and some other stuff.
Everything old is new again #
Thanks to Hugo and the Hugo-Octopress theme, this blog now looks a lot like it originally did. Of course, I found it necessary to fork and expand on Octopress, so I’ve had to do that again to fully support my old blog posts. Luckily Hugo shortcodes are pretty easy and convenient.
codeblock #
The codecaption
shortcode
from the Hugo Octopress theme gives a nice Octopress look, but I really like to specify a starting
line number with a link to that line in the source repository. So along with a title, I need a
URL, the link text, and optionally a line number on which to start.
|
|
rawhtml #
I also tripped over how to handle the HTML I’d embedded directly in a couple of blog posts. Those sections would simply not show up at all, unless I opted for “unsafe” rendering in the markdown settings. Instead of doing that, I found someone’s trivial shortcode to wrap around them:
|
|
Close enough #
I’m sure I’ll tweak more in future (maybe even fork the theme), but so far I’m satisfied.
Beholder on Your Phone pt6 #
At some point during my hiatus, I resumed work on the SMS port of Beholder High. I was close to a textual MVP, but the art was one of the best parts of the game. The web-based version of the game was free to composite the images in real time, and do some idle animations; but for the sake of bandwidth and simplicity, I decided it was better to pre-process the images for the SMS (technically SMS and MMS, now) version.
A Little Image Magick #
I had some history with ImageMagick, so was glad to find a handy Ruby gem: rmagick. With some trial and error, I started putting together images in MMS-friendly resolution:
|
|
I wasn’t going to spend much time putting together tests for this run-once code, so the ability to superimpose debug info on the image was useful for human verification.
|
|
Continuous (Visual) Integration #
Of course I wanted to generate the images as part of a CI workflow, which seemed straightforward:
|
|
…but had issues with my debug text.
$ bundle exec ruby image_comp.rb
/usr/local/bundle/gems/json-2.1.0/lib/json/common.rb:156: warning: Using the last argument as keyword parameters is deprecated
image_comp.rb:38:in `annotate': unable to read font `Helvetica' @ error/annotate.c/RenderFreetype/1396 (Magick::ImageMagickError)
from image_comp.rb:38:in `debug_text'
from image_comp.rb:17:in `beholder_compose_image'
from image_comp.rb:56:in `block in <main>'
from image_comp.rb:47:in `each'
from image_comp.rb:47:in `<main>'
After a little research, it seemed like maybe installing Ghostscript would give me the fonts I needed, so I added that.
|
|
I ran the image compositing at part of the rspec test pipeline, and archived the results.
|
|
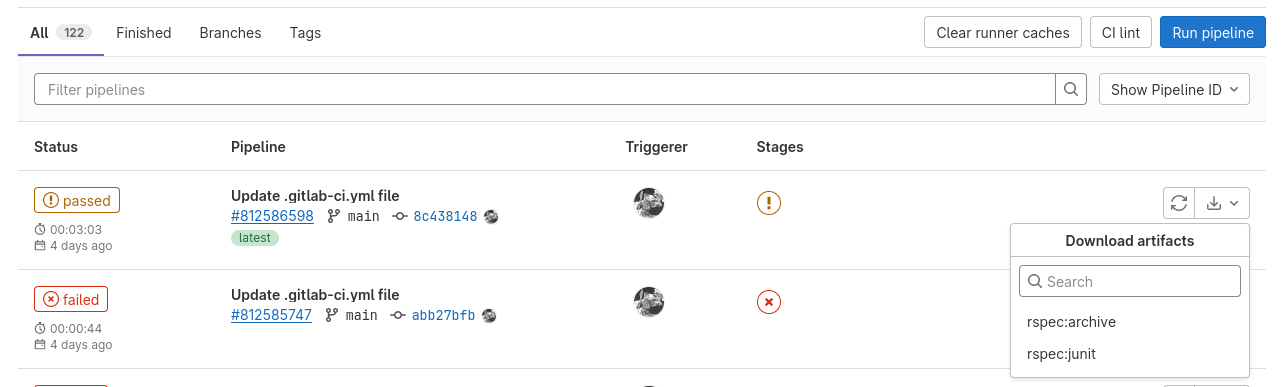
Download and unzip, and…
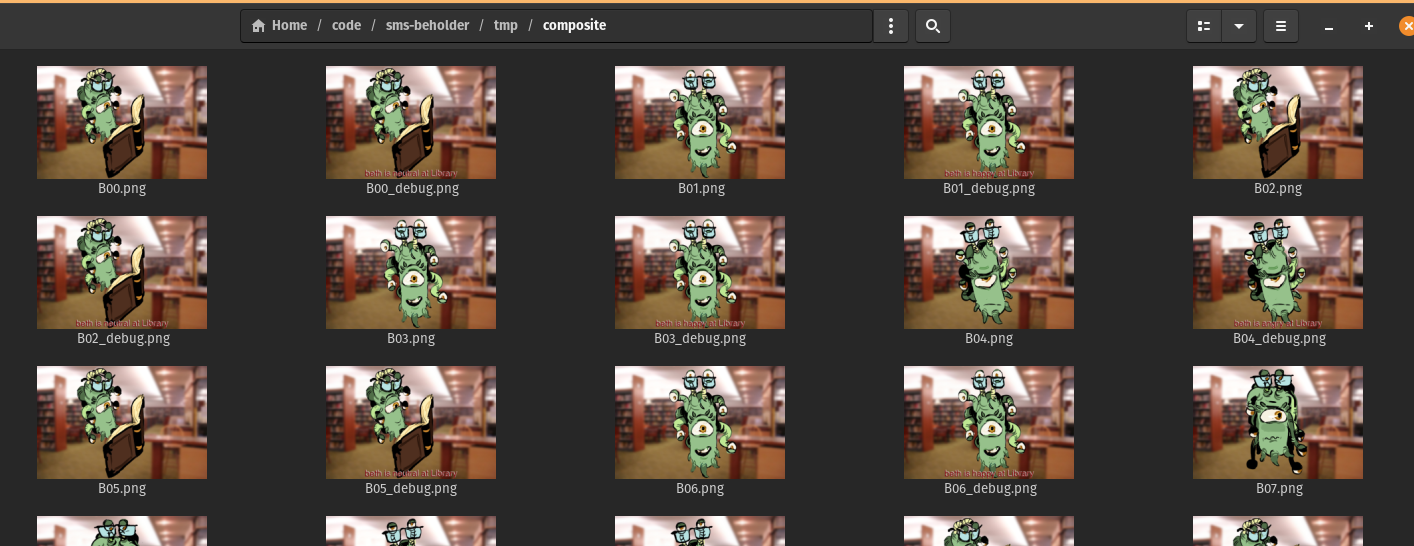
Now to put them somewhere public so they can be attached to messages.