Tests, Part 1 of NaN.
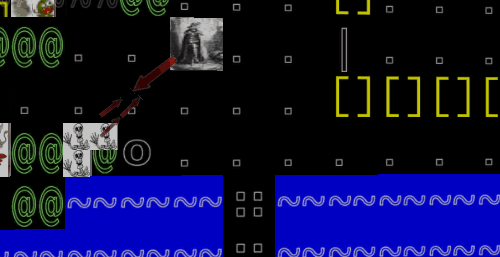
Coding #
Ok, time to start moving forward on the whole “Let’s wrap this thing in tests so we can safely replace all of the tech” thing. QA terminology varies between industries, between groups in the same industry, between people in the same group even; but I like to call this kind of stuff “arm’s length” testing. Tests run, or can run, on a separate machine from the code being tested, and are run on a “release” build. It’s pretty natural for client-server systems, where we’ll just pretend to be a normal client (for the most part).
Let’s start with a Cucumber feature file:
|
|
And some definitions for those steps:
|
|
and we’re off to the races, once we define random_char_name
.
|
|
To make sure I’m getting valid names, I started with code from the server’s CharGen.cs
;
specifically, CharacterNameDenied
. But then why am I calling it with max_length
of 10, and
why no period in the acceptable
array? Because I immediately ran into issues with the account
name being rejected. A little digging found there were different rules there.
|
|
|
|
So I’ll have to treat the various parts of the user a little differently. But for now, does this work?
|
|
…
|
|
Hooray! Crude, but effective. A start.
A note about Cucumber #
It looks like the online docs are being rewritten as we speak. I found saving a copy of the
output of cucumber --help
to be, well, helpful.
Playing #
The Talos Principle #
Second playthrough, about to try exploring the dialog trees now that I’ve done all of the main puzzles again.
Tearaway: Unfolded #
Having played the original on the Vita, played through mostly to see the differences and to experience that world on the big screen. Even if you haven’t/won’t play it, go here for a glimpse of how it was built.