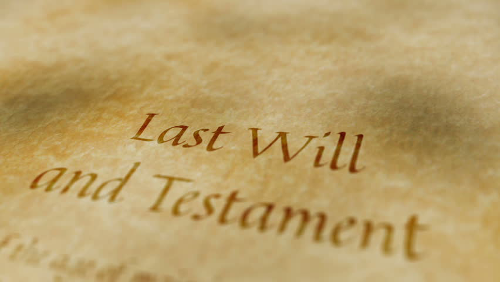
In which we start converting to plain(ish) Jekyll in earnest, and do the other things too.
Test Every Day #
Setting aside the more involved “Combat damage in-game” task for the moment, what’s the next thing
to test? Ah, UnderworldEnabled
. If it was in the original game, it was definitely added after my
time. Let’s see what the config option does.
|
|
and there are a few ways to get to this code:
- Speaking the Ancestor Start Chant (“ashak ashtug nushi ilani”) in the ancestor start cell
- Speaking the Underworld Portal Chant (“urruku ya zi xul”) in the underworld portal cell
- Your corpse is burned while you’re still online and with positive karma
- Your corpse is burned while you’re still online and of Evil alignment, and fail a constitution saving throw
- You are older than “ancient” and lose a die roll (roughly 1/20 chance per round)
- Rest when dead with positive karma, in a map with no karma res point, if not a thief
- Rest when dead but not lawful, in a map with no karma res point, if not a thief
Ancestor Start #
Given that characters in IoK did actually age and die, “ancestoring” was introduced as a way to pass down some of a player’s accomplishments to a new character. Like so many other things, it had to be done at a particular site with a particular set of magic words.
So how do we mark a map cell as an Ancestor Start cell?
|
|
So it’s baked into the map file. Easy enough to update the Test01
map to include it. We’ll throw
in an Ancestor Stop and Underworld Portal cell while we’re at it:
<z>0</z> <x>40</x> <y>25</y> <f>light</f> <n>Test01 Surface</n> outdoor=true
WWWWWWWWWWWWWWWWWWWWWWWWWW
WW. . . . . . . . . . . WW
WW. . . . . . . . . a0. WW
WW. . . . . . . . . . . WW
WW. . . . . . . . . a1. WW
WW. . . . . . . . . . . WW
WW. . . . . . . . . gg. WW
WW. . . . . . . . . . . WW
WW. . . . . . . . . . . WW
WWWWWWWWWWWWWWWWWWWWWWWWWW
Ok, let’s try these two easy tests:
|
|
Building on what we already have, there’s almost zero significant code to be written for these. Even moving the player (in the database, while they’re not logged in) is simple:
|
|
TODO/Refactor Every Other Day #
The big TODO in the test code that leaps out at me today is the verbosity of the output. I’ve been remiss, leaving in raw debug output when the code is done.
The output from running the current 34 scenarios with debug off is 1480 lines. (With debug on, it’s
3880.) Let’s abstract out a debug_msg
method and search for all of the puts
s. We’ll retool the
telnet output as well while we’re at it.
|
|
(Note: I also changed DEBUG
to TEST_DEBUG
because it was putting the server in debug mode,
which was not the intention.)
So let’s see how chatty we are now:
- TEST_DEBUG false, TELNET_TRACE false: 357 lines.
- TEST_DEBUG false, TELNET_TRACE text: 2930 lines.
- TEST_DEBUG true, TELNET_TRACE html: 3112 lines.
- TEST_DEBUG true, TELNET_TRACE html: 4038 lines.
That seems a bit better, and paging through the non-debug output shows me only what Cucumber has to say.
Improve The Blog Itself Every Third Day #
I finally found a Jekyll theme I can make a good start with, the Jekyll Dark Clean Theme. I tweaked it a bit and converted the first few days. More work is needed, but I think I’ll be able to switch to it soon.
It was surprisingly difficult to find a theme that fulfilled my requirements: Codeblocks that work well (with line numbers), multiple tags on a post, easily inserted images (that render correctly), and title and/or side bars that were not intrusive.
Useful Stuff #