Time to reactivate the database code and see if we can get a less static scene…
Talking to the ‘Base #
In order to get things up and running without having to deal with it, I had commented out the database calls in the code I was leveraging to load the map. I have a running instance of Dragon’s Spine 2.0.16.5 and the appropriate Microsoft SQL Server database set up, so now I can tackle reading from it.
Once I started re-enabling code, I had to copy over the appropriate Mono version of System.Security.dll. But still nothing works. Time to kludge the ornate logging code into spitting things out to my Unity console:
public static void LogException(Exception e)
{
// Tell the Unity console about it:
Debug.Log(e.Message.ToString());
Debug.Log(e.StackTrace.ToString());
public static void Log(string message, LogType logType)
{
// Tell the Unity console about it:
Debug.Log(message);
So then I can see “SQL Server does not exist or access denied”. Time to start troubleshooting my (hardcoded, for now) connection string.
Try a deliberately incorrect password, get the same error. Bad user ID, same.
Bad instance name in “Data Source”, same. Bad server name in “Data Source”, then “No such host is known”. So we’re having trouble with the instance.
But the Dragon’s Spine executable I build in Visual Studio is hitting that instance just fine. After a lot of lost time trying to understand SQL Server logging, I tried starting a service that wasn’t running:

…and suddenly all was well. I guess the native .NET magic Visual Studio was giving me didn’t need the Browser service to hit something on localhost, but the Mono stuff in Unity did.
To be on the safe side, we’ll need a read-only user in SQL Server. The internet is useful as always. I added the user but then was denied the ability to EXECUTE the necessary stored procedure, so I had to add access to that specifically.
Cell Descriptions #
The obvious thing is to try the map cells first. Near the end of Map.LoadMap:
// load the cell information from the Cell table
List<Cell> cellsList = DAL.DBWorld.GetCellList(this.Name);
// The above was previously commented out in favor of:
// List<Cell> cellsList = new List<Cell>();
string key = "";
foreach (Cell iCell in cellsList)
{
key = iCell.X.ToString() + "," + iCell.Y.ToString() + "," + iCell.Z.ToString();
if (this.cells.ContainsKey(key))
{
this.cells[key].Segue = iCell.Segue;
this.cells[key].Description.Trim();
this.cells[key].Description = iCell.Description + " " + this.cells[key].Description;
this.cells[key].Description.Trim();
this.cells[key].cellLock = iCell.cellLock;
this.cells[key].IsMapPortal = iCell.IsMapPortal;
this.cells[key].IsTeleport = iCell.IsTeleport;
this.cells[key].IsSingleCustomer = iCell.IsSingleCustomer;
}
}
and we add some debug to our map loading code to see if we’re getting any of it:
foreach (string k in cellKeyList) {
Vector3 mapPosition = new Vector3(ourMap.cells[k].X, ourMap.cells[k].Y, ourMap.cells[k].Z);
cells[mapPosition] = ourMap.cells[k];
cellNeedsTransform[mapPosition] = true; // Every cell needs a transform by default
if (ourMap.cells[k].Description != "") {
print(mapPosition + " description is " + ourMap.cells[k].Description);
}
}
Nothing, until I realize I need to give the map a name that matches the DB:
ourMap = new Map();
ourMap.Name = "Island of Kesmai";
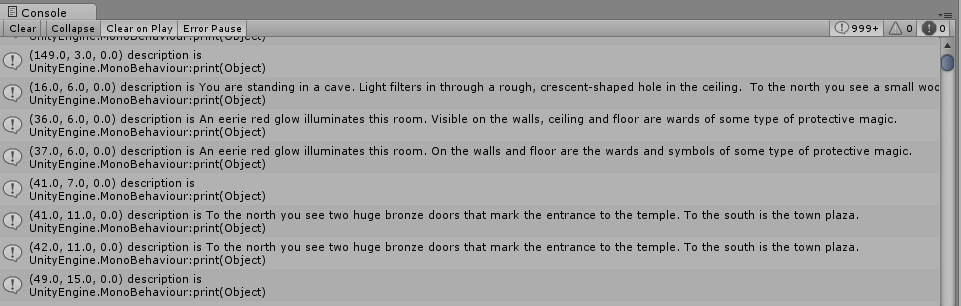
Portals #
Just to tie something visual to the database-driven cell data, let’s show some particle effects where we know there are portals. As we’re iterating through the cells…
if (cells[v].IsMapPortal) {
Vector3 position = new Vector3((-1f) * cells[v].X, (-1f) * cells[v].Y, (0.1f * cells[v].Z) + 0.5f);
Transform tempts = Instantiate(portalPrefab,
position,
Quaternion.identity) as Transform;
}
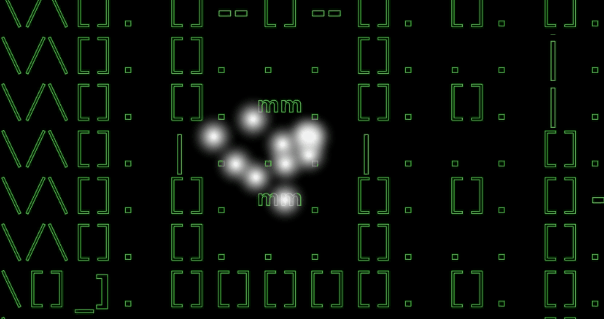
Pretty, yes, but still not really “live”. That’ll take a bit of doing.