Finally switching between maps on the fly.
Apologies #
Sorry for the long delay between posts; epic week at work.
Coding #
Beyond map 3 #
In the table that stores the Cell data for a map, I had foolishly included the landID
field (it indicates whether a given map belongs to the
Beginners’ Game, Advanced Game, or Underworld) which is always the same for a given mapID
. Hard-coding landID
to zero just gave me the
first four maps, so I modified the query in the stored procedure to ignore the field entirely.
|
|
And of course needed to modify the call in the code to match.
|
|
Ditto NPCs. So now we can easily pull from any map we want.
Switching maps #
The managers need to handle switching between maps. We’ll start them out on map “-1” and add a setter and coroutine in a by-now familiar pattern.
|
|
|
|
The code that was originally only called once has moved into InitialMapLoading
, and we clear everything out (except the object pool and material dictionary)
before calling it for the new map. You may notice a lock
which needed to be added; in the MapManager
we are sharing the various cell structures with the DB
access thread, so we need some safety.
Something very similar was done in NpcManager
, minus the locks because we’re more safely passing lists around there.
Material Caching #
Map-changing performance was initially quite bad on some maps, until I switched the NpcManager
over to keeping a dictionary of Materials that persisted.
|
|
I had been doing this in MapManager
the whole time, but was kind of surprised at the impact that using it in NpcManager
had. I guess calls to Resources.Load
are really as expensive as you’d think.
Defining some vantage points #
Just to make sure we have a good view of each new map when we switch to it, I manually added an initial camera position for each via the editor.
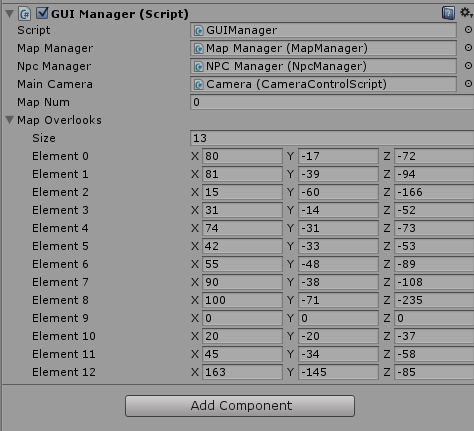
Note that these would change between aspect ratios; I did these for 16:9.
And a keypress to cycle #
|
|
And some new Materials #
For cell types that didn’t exist on the first few maps.
All for a cool gif #
(Pauses edited out for your enjoyment. We can’t really load new maps that fast. Yet.)
Well, not just for this cool gif. Now we have all of the plumbing in place to switch maps on the fly, in search of action or to follow a character going through a portal or whatever.